App Verify API - Review app best practices
NOTE:
To add this product to your account, contact a Telesign expert. This product is available for full-service accounts only.
This page discusses all the best practice to take into consideration for your application when designing it.
Ensure secure communication
For best effect, ensure that end user devices communicate to your server securely when initiating the App Verify API flash call. Do not store your API key on the application for communication with Telesign.
Verify the code before finalizing
You should make sure to verify the code received from the call to the device on your server before calling finalize. Avoid sending the verification code to the device for validation.
Prompt user to enter phone number of mobile device
Telesign recommends that you show a message prompting end users to enter the mobile phone number of the device on which the application is downloaded and installed, not the number of another device or landline. For example, the message might read:
"Please enter the mobile phone number of the device on which this application is installed and make sure that the cellular connection is on."
You should attempt auto verification only on mobile devices capable of receiving a circuit-switched voice call. For example, if an end user downloads your app to a tablet that can perform only data traffic, auto verification will fail.
In cases where the end user does not want to provide a mobile number, you should design your application workflow appropriately to utilize other types of non-mobile verification (such as Voice Verify API or SMS Verify API) to provide the user with an option for an alternate form of verification, along with an appropriate message.
Enter country and phone number separately
Your application should display the country code (or country name) and the phone number in separate input text boxes. Recommended layouts are to position the fields vertically on different lines, or to position them horizontally with a gap between them. Often when end users enter the country code and phone number in the same field, it results in mistakes such as users adding a preceding '0' or '+', or inserting a '0' between country code and phone number, as practiced in different countries.
Auto-extraction
Telesign recommends that your application not auto-extract phone numbers. Programmatic auto-extraction of phone numbers does not work consistently for various service providers and locales. If the auto-extraction support of phone number is provided, it is recommended that developers provide an option for the end users to edit the phone number.
Auto extraction does not work well in every country, however, it can be a nice feature to offer in your application. Auto-extraction of a phone number works reliably (80% of the time or more) in many countries.
Notify the end user they will receive a voice call
Make sure your end user is aware that they will receive a voice call for verification purposes and that they should ignore it.
Recommended flow for missed call verification
The following is a best practice for user experience that provides the recommended flow for missed call verification.
- Your sample app should prompt the end user for their phone number. You should keep country code and phone number separate in the display. The field for entering the phone number should not allow special characters or spaces.
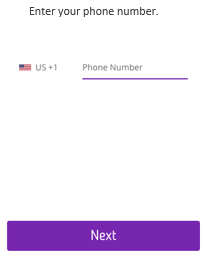
- After the end user presses Next, prompt them with a screen explaining how they will be verified and what you want them to do. The bottom of the screen should offer them the choice to retry if the call did not happen, or a choice to continue if they receive the call.
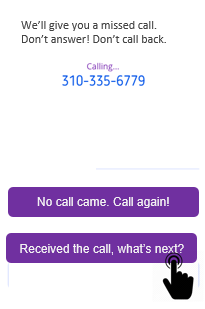
- Ask the end user to retrieve the last digits of the number they were called from. You can create a display that shows the number not completed to help them visually understand what you want them to do. In this example, th end user needs to fill in the empty spaces.
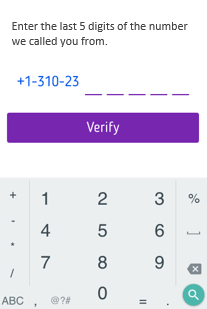
- This screen demonstrates what it might look like when the end user goes to retrieve the verification code from the missed call.
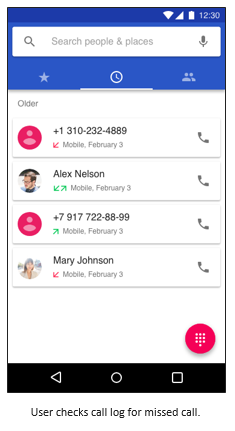
- This screenshot shows you what it would look like when you have the end user enter the verification code. When they are done inputting the code, they press a button to kick off the verification process.
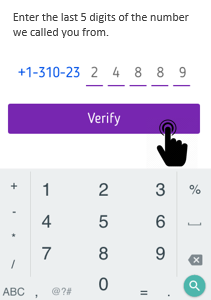
- If everything goes well, the verification is a success. You can continue with any next steps in your app.
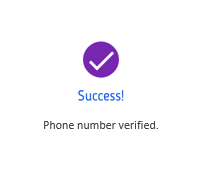
Updated 3 months ago