SMS Verify API - Tutorial: Send one-time passcode with Telesign Python SDK
This tutorial teaches you how to use the Telesign Python SDK to send an SMS with a one-time passcode (OTP). Go to GitHub to see the complete sample code.
Before you begin
Make sure you have the following before you start:
- Authentication credentials: Your Customer ID and API Key. If you need help finding these items, go to the support article How do I find my Customer ID and API Key.
- Testing device: A mobile phone on which you can receive SMS.
NOTE:
- This tutorial uses a Mac. Please modify accordingly if you are using a different system.
- This tutorial uses Python 3.8.1. Please modify accordingly if you are using a different version of Python.
Set up your project
CAUTION:
You should use the Full-service SDK for SMS Verify API even if you have a Self-service account!
-
Follow the Telesign Full-service Python SDK install instructions on GitHub here, incorporating the following details:
- Use
sms_verify
as the project name.
You should end up in the top-level directory (
sms_verify
) for your project in the Terminal. - Use
-
Create a new file called "verify_with_own_code.py" and open it in your editor.
touch verify_with_own_code.py
Create code to send the SMS
-
Open the file "verify_with_own_code.py".
-
Import dependencies from the Telesign Self-service and Full-service SDKs.
from telesignenterprise.verify import VerifyClient from telesign.util import random_with_n_digits
-
Import a package for working with environment variables.
import os
-
Define variables to store your authentication credentials. For testing purposes, you can just overwrite the second parameter of each
getenv
function call below with your credentials or use environment variables.customer_id = os.getenv('CUSTOMER_ID', 'FFFFFFFF-EEEE-DDDD-1234-AB1234567890') api_key = os.getenv('API_KEY', 'ABC12345yusumoN6BYsBVkh+yRJ5czgsnCehZaOYldPJdmFh6NeX8kunZ2zU1YWaUw/0wV6xfw==')
-
Define a variable to hold the end-user's phone number you want to send an OTP to. For this tutorial, hardcode your testing device's phone number or pull it from an environment variable.
phone_number = os.getenv('PHONE_NUMBER', '11234567890')
NOTE:
In your production integration, pull the phone number from your recipient database instead of hardcoding it.
-
Randomly generate your OTP. We will use a Telesign SDK utility for this. The parameter value
5
specifies the number of digits generated:verify_code = random_with_n_digits(5)
CAUTION:
The method used above to generate a code is actually pseudo-random. In your production implementation, you might want to use a more robust method for randomizing.
-
Instantiate a verification client object with your authentication credentials.
verify = VerifyClient(customer_id, api_key)
NOTE:
When you use a Telesign SDK to make your request, authentication is handled behind-the-scenes for you. All you need to provide is your Customer ID and API Key. The SDKs apply Digest authentication whenever they make a request to a Telesign service where it is supported. When Digest authentication is not supported, the SDKs apply Basic authentication.
-
Make the request and capture the response. Behind the scenes, this sends an HTTP request to the Telesign Verify API. Telesign then sends an SMS with an OTP to the end-user.
response = verify.sms(phone_number, verify_code=verify_code)
-
Display the response in the console for debugging purposes. In your production code, you would likely remove this.
print(f"\nResponse HTTP status:\n{response.status_code}\n") print(f"\nResponse body:\n{response.body}\n")
-
Collect the asserted OTP entered by the end-user in your application. You can simulate this by prompting for input from the command line.
user_entered_verify_code = input("Please enter the verification code you were sent: ")
NOTE:
In your production implementation, collect input from your website or other application where the end-user is trying to sign in.
-
Determine if the user-entered code matches your OTP, and resolve the sign in attempt accordingly. You can simulate this by reporting whether the codes match.
if verify_code == user_entered_verify_code.strip(): print("Your code is correct.") else: print("Your code is incorrect.")
NOTE:
In your production implementation, add code here to sign in the user when the user-supplied code matches the OTP.
Test your integration
-
Switch from your editor to the terminal and run "verify_with_own_code.py".
python verify_with_own_code.py
You should receive an SMS on your phone that looks like this:
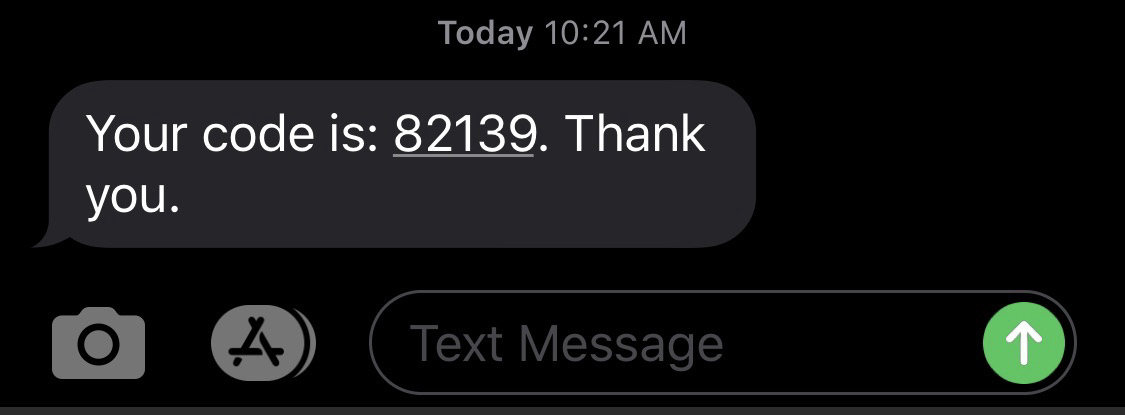
-
Enter the OTP you received on your phone at the command prompt on the terminal to test that verification is successful:
Please enter the verification code you were sent: 82139 Your code is correct.
-
Now let's test an unsuccessful verification. Run again.
python verify_with_own_code.py
You should receive a new OTP on your phone.
-
Enter something else that isn't correct at the command prompt on the terminal and you should get a message that verification failed:
Please enter the verification code you were sent: 55555 Your code is incorrect.
Sample code
The complete sample code for this tutorial can be found on GitHub.
Updated about 1 year ago