Verify API - Security implementation details for Push Verify callbacks
This page contains the security implementation details you need to know for the Push Verify callbacks webhook.
Generate the key
- Secret: A predefined secret stored securely on the server.
- Correlation ID: A unique identifier for each transaction.
- Timestamp: The time the message is sent to ensure its recency.
The key is generated by concatenating these values and then using a SHA-256 hash function to secure them.
import hashlib
import time
def generate_key(secret, correlation_id):
timestamp = str(int(time.time()))
raw_key = f"{secret}{correlation_id}{timestamp}"
return hashlib.sha256(raw_key.encode()).hexdigest(), timestamp
Send the key with the push message
When sending the push notification through Firebase, include the generated key and timestamp in the message payload. This allows the client (either Telesign's SDK or the customer's app) to use these values to compute the HMAC.
HMAC creation on the client side
The client will use the received key to create the HMAC signature of the message status.
import hmac
def create_hmac(message, secret_key):
return hmac.new(secret_key.encode(), message.encode(), hashlib.sha256).hexdigest()
Verify HMAC on the webhook
When the webhook receives the message status with the HMAC, it should:
- Recompute the HMAC using the same method as the client.
- Compare the recomputed HMAC with the one received.
- Ensure the timestamp is within an acceptable range to prevent replay attacks.
def verify_hmac(received_hmac, message, secret_key):
expected_hmac = create_hmac(message, secret_key)
return hmac.compare_digest(received_hmac, expected_hmac)
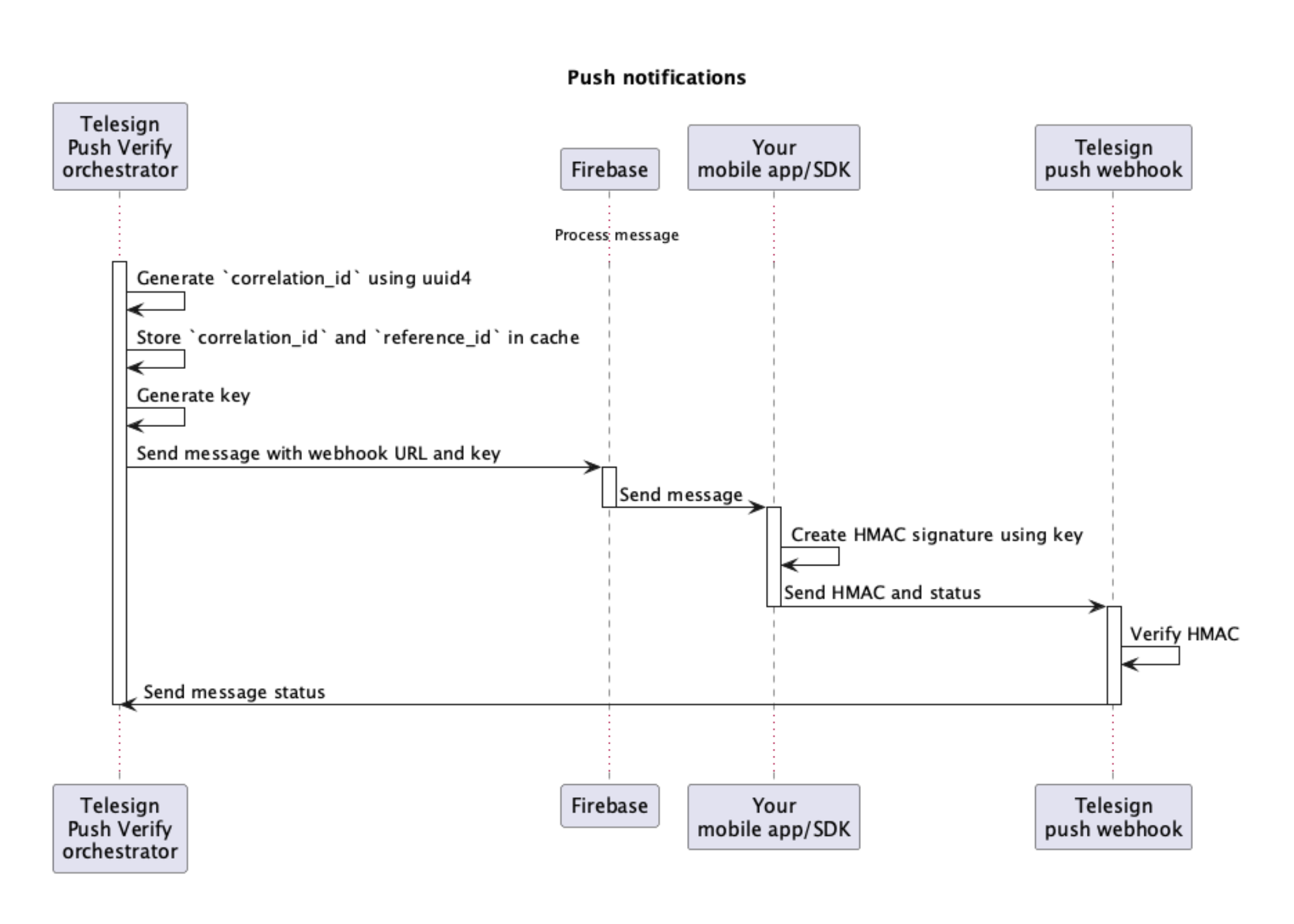
Updated 8 days ago